type HelloRequest { name:string } interface HelloInterface { requestResponse: hello( HelloRequest )( string ) } service HelloService { execution: concurrent inputPort HelloService { location: "socket://localhost:9000" protocol: http { format = "json" } interfaces: HelloInterface } main { hello( request )( response ) { response = "Hello " + request.name } } }
Thinking in services
Jolie crystallises the programming concepts of service-oriented computing as linguistic constructs. The basic building blocks of software are not objects or functions, but rather services that can be relocated and replicated as needed. A composition of services is a service.
Get Started Now
Follow this tutorial to start your first Jolie project.
Tailored for microservices and APIs
Jolie is a contract-first programming language, which puts API design at the forefront. It supports both synchronous and asynchronous communication. Data models are defined by types that support refinement (in red on the right), and DTO (Data Transfer Objects) transformations are transparently managed by the interpreter.
type GetProfileRequestType { id:int } type GetProfileResponseType { name:string surname:string email:string( regex(".*@.*\\..*") ) accounts[0,*] { nickname:string service_url:string enabled:bool } ranking:int( ranges( [1,5] ) ) } type SendMessageRequestType { id:int message:string( length( [0,250] ) ) } interface ProfileInterface { requestResponse: // Synchronous RPC getProfile( GetProfileRequestType )( GetProfileResponseType ) oneWay: // Asynchronous sendMessage( SendMessageRequestType ) }
Built for the networked age
Jolie code is always contained in services, which you can always move from being local to remote and vice versa, without altering the logic of your programs. A monolithical application can scale to being distributed by design: if you decide to distribute a part of it, just take it and execute it in another machine.
Program your microservice system
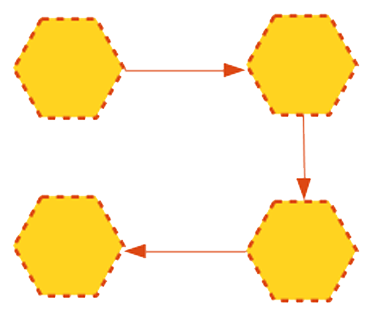
Deploy it in a single machine
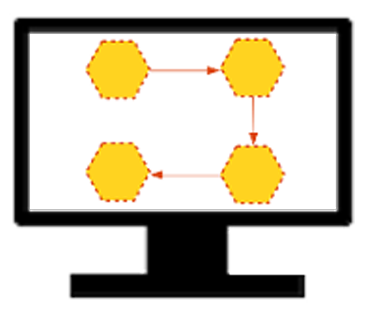
Deploy it in two different machines
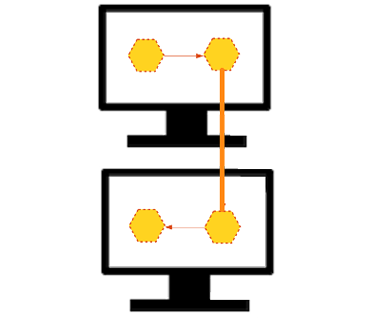
Deploy it in four different machines
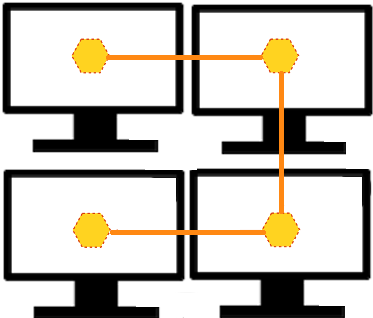
Protocol agnostic
Jolie is protocol agnostic: your services can exchange data by using different protocols. Bridging two networks using different protocols is a matter of a few lines of code. If you need a protocol that Jolie does not support yet, there is an API for developing new ones in Java.
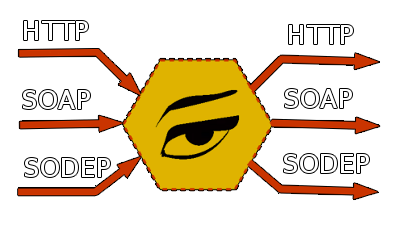
type HelloRequest { name:string } interface HelloInterface { requestResponse: hello( HelloRequest )( string ) } service HelloService { execution { concurrent } inputPort HelloServiceHTTP { location: "socket://localost:9000" protocol: http { format = "json" } interfaces: HelloInterface } inputPort HelloServiceSOAP { location: "socket://localost:9001" protocol: soap { wsdl = "myWsdl.wsdl" wsdl.port = "MyServiceSOAPPort" } interfaces: HelloInterface } inputPort HelloServiceSODEP { location: "socket://localost:9002" protocol: sodep interfaces: HelloInterface } main { // This can now be reached through HTTP/JSON, SOAP, or SODEP (binary) hello( request )( response ) { response = "Hello " + request.name } } }
Structured workflows
Jolie comes with native primitives for structuring workflows, for example in sequences (one after the
other) or parallels (go at the same time). This makes the code follow naturally from the requirements,
avoiding error-prone bookkeeping variables for checking what happened so far in a computation. For
example, the following code says that the operations publish
and edit
become
available at the same time (|
), but only after (;
) operation
login
is invoked:
login( credentials )() { checkCredentials }; { publish( x ) | edit( y ) }
Dynamic error handling for parallel code
Programming reliable parallel code is challenging because faults may cause side-effects in parallel
activities. Jolie comes with a solid semantics for parallel fault handling. Programmers can update the
behaviour of fault handlers at runtime, following the execution of activities thanks to the
install
primitive.
include "console.iol" include "time.iol" main { scope( grandFather ) { install( this => println@Console( "recovering grandFather" )() ); scope( father ) { install( this => println@Console( "recovering father" )() ); scope ( son ) { install( this => println@Console( "recovering son" )() ); sleep@Time( 500 )(); println@Console( "Son's code block" )() } } } | throw( a_fault ) }
Everything you build can be used to build again
Jolie offers many ways for building complex software from simple services. Even the deployment architecture of a system can be programmed with native primitives, generalising common practices. Whatever you build, is again a service that you can expose; so, it can be reused to build again! Here are some examples of composition:
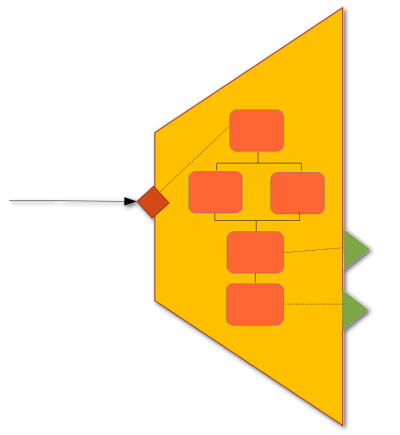
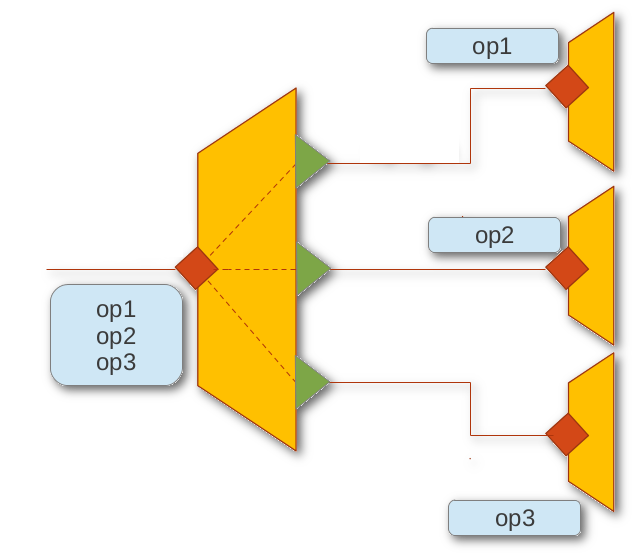
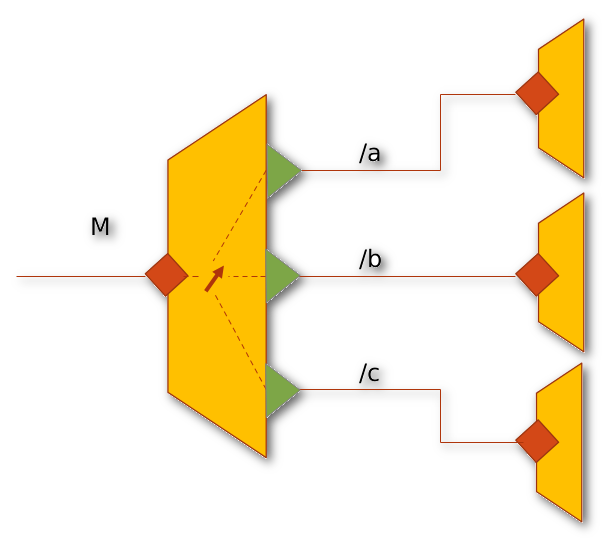
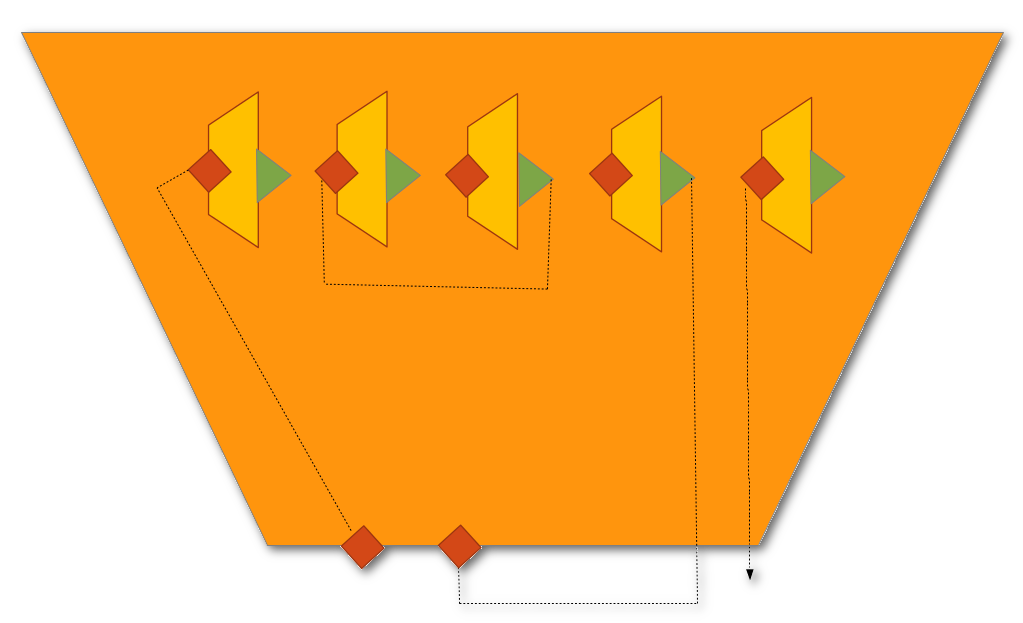
Revolutionise the way you develop web applications
Jolie allows for directly programming a web server that is just a service as everything else in Jolie. You don't need other extra frameworks for instantiating a web server.
It is possible to directly aggregate other service APIs into a Jolie web server, thus web GUIs are completely independent from the microservice system they interact with. We natively support JSON, XML, AJAX, ND-JSON and other technologies.
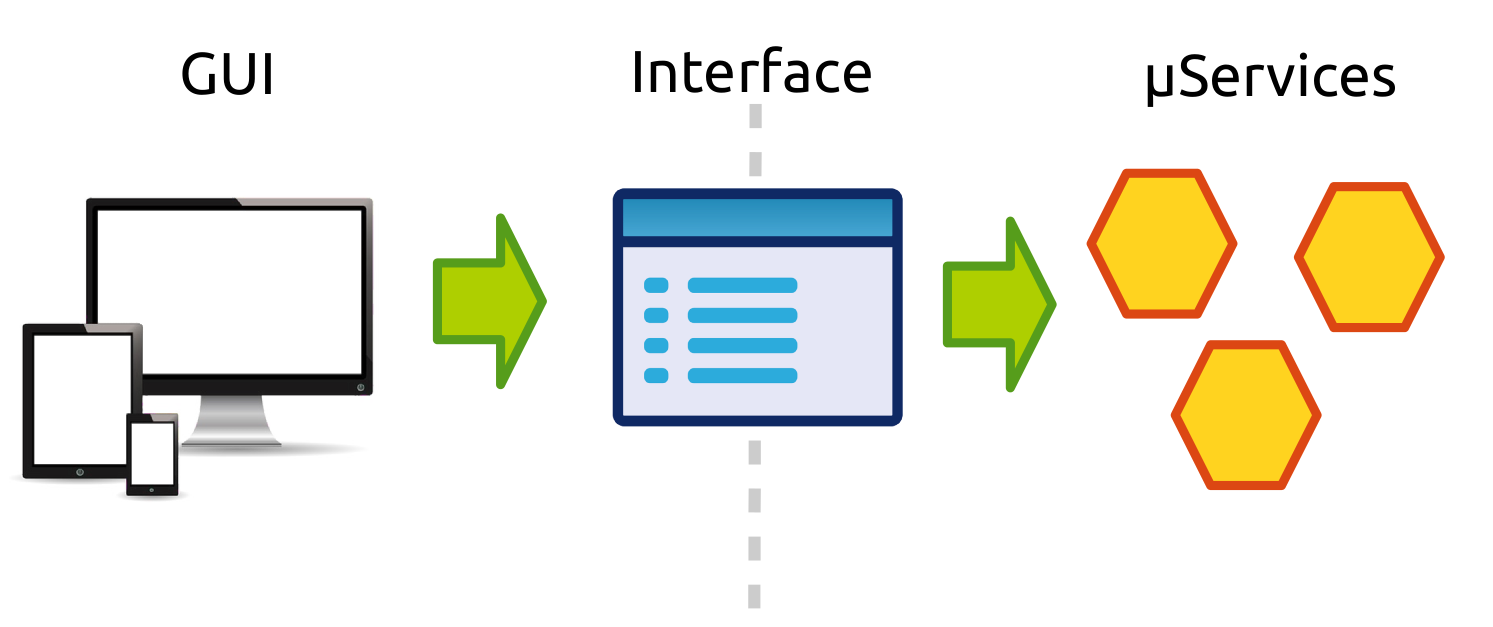
Solid foundations
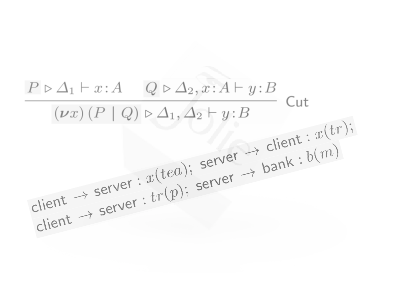
Join us!
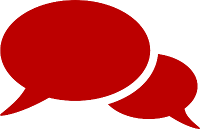